I recently came across a very interesting puzzle, I will be happy to share my thoughts:
In the given code snippet, if you remove Line-2, the result of Line-4 will change from false to true. Let’s dive into why this happens.
String string1 = "Hello".concat("World"); // Line-1
String string2 = new String("HelloWorld"); // Line-2
String string3 = string1.intern(); // Line-3
System.out.println(string1 == string3); // Line-4
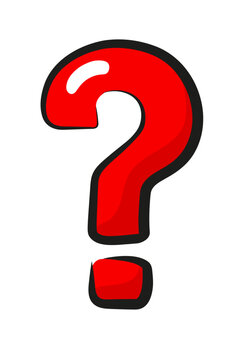
Line-1: Here, string1 is created by concatenating two strings: “Hello” and “World”. The result is a new String object representing “HelloWorld”. There is no internment here, only two objects “Hello” and “World” are created on the constant pool because they are created using literals.
Line-2: In this line, string2 is created by explicitly constructing a new String object with the content “HelloWorld”. It’s important to note that this is a separate String object and not related to string1. Two objects are created here, one from a literal “HelloWorld” and goes to the constant pool and the other by new and placed on the heap.
Line-3: The intern() method is called on string1. This method checks if a String with the same content as string1 (“HelloWorld”) exists in the constant pool. If it does, it returns a reference to that existing String, otherwise, it adds string1 to the constant pool and returns a reference to it. In this case, “HelloWorld” is already in the constant pool (represented as string2), so string3 gets a reference to the same String object as string2.
Now, if you remove Line-2, both string1 and string3 will point to the same String object, which is the result of concatenating “Hello” and “World.” Consequently, the comparison string1 == string3 in Line-4 will return true because both variables now reference the same object in memory, which was interned in Line-3.
These are my thoughts on this puzzle, if someone has a better explanation, please discuss it in the comments.