For the past week, I’ve been learning the basics of Angular. With some free time, I went through the tutorial on www.angular.dev and did my own exercises. I created new components and a service, which I later used across different components. The initial version of the app was simple, with a hardcoded array of employee objects. I display processed employee data in various ways on the view. It was a great syntax training of *ngFor and @for.
I tried to enhance my app by fetching employee data from a separate JSON file using the HttpClient for learning purposes. However, I faced a small problem. Everything seemed correct, but the data wasn’t displaying. I checked the JSON file URL and my employee.service.ts injection, and everything seemed fine. Analyzing the console, I found a surprise error:
main.ts:6 NullInjectorError: R3InjectorError(Standalone[_AppComponent])[_EmployeeService -> _EmployeeService -> _HttpClient -> _HttpClient]: NullInjectorError: No provider for _HttpClient! // ... more details
I now knew where to look. Something was wrong with HttpClient – maybe a missing import? I asked ChatGPT, Bard, and Bing for help, but the responses were confusing and unhelpful. After spending hours on the problem, I almost lost hope.
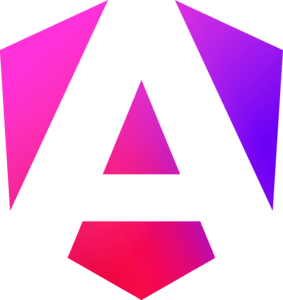
Then, I found a similar issue on StackOverflow with a suggested solution:
providers: [provideHttpClient()]
I dug deeper and realized I needed to add this in my file:
import {provideHttpClient} from '@angular/common/http';
export const appConfig: ApplicationConfig = {
providers: [ provideHttpClient()]
};
Adding this brought an immediate smile to my face. It solved the problem I struggled with for hours.
As a bonus, I discovered another tool – json-server. It helped me simulate a fake API from my JSON file:
npm install -g json-server
json-server --watch myDB.json
Now, at http://localhost:3000/, we have a fake API from our JSON file!